Create Nested Permissions
One of the greatest benefits of GraphQL is the ability to perform nested queries across types — all from a single query! For this, you can also implement permissions.
Imagine wanting to execute the following query:
query GetUsers {users {idnameorders {idcreated_atstatusdelivery_date}notifications {idmessagecreated_atupdated_at}}}
In order to do this, we'll need to set up permissions for the orders
and notifications
models.
Orders
ModelSelectPermissions
Begin by finding the ModelPermissions
for your orders
table:
kind: ModelPermissionsversion: v1definition:modelName: orderspermissions:- role: adminselect:filter: null
We'll add a filter to compare the x-hasura-user-id
session variable to the user_id
field on the orders
table.
The complete ModelPermissions
for orders
should look like this:
kind: ModelPermissionsversion: v1definition:modelName: orderspermissions:- role: adminselect:filter: null- role: userselect:filter:fieldComparison:field: user_idoperator: _eqvalue:sessionVariable: x-hasura-user-id
TypePermissions
You can search for the TypePermissions
for your orders
table:
kind: TypePermissionsversion: v1definition:typeName: orderspermissions:- role: adminoutput:allowedFields:- created_at- delivery_date- id- is_reviewed- product_id- status- updated_at- user_id
We'll let the user see all fields for their orders, so our final TypePermissions
for orders
should look like this:
kind: TypePermissionsversion: v1definition:typeName: orderspermissions:- role: adminoutput:allowedFields:- created_at- delivery_date- id- is_reviewed- product_id- status- updated_at- user_id- role: useroutput:allowedFields:- created_at- delivery_date- id- is_reviewed- product_id- status- updated_at- user_id
Notifications
We'll repeat the process for our notifications
table:
ModelSelectPermissions
After modifying the ModelPermissions
for your notifications
table, it should look like this:
kind: ModelPermissionsversion: v1definition:modelName: notificationspermissions:- role: adminselect:filter: null- role: userselect:filter:fieldComparison:field: user_idoperator: _eqvalue:sessionVariable: x-hasura-user-id
TypePermissions
Finally, we'll modify the TypePermissions
for our notifications
table:
kind: TypePermissionsversion: v1definition:typeName: notificationspermissions:- role: adminoutput:allowedFields:- created_at- id- message- updated_at- user_id- role: useroutput:allowedFields:- created_at- id- message- updated_at- user_id
Test the new permissions
Then, run the query in the Console, ensuring that you have the x-hasura-role
and x-hasura-user-id
headers set:
query GetUsers {users {idnameorders {idcreated_atstatusdelivery_date}notifications {idmessagecreated_atupdated_at}}}
You'll see that you can only see the orders and notifications for the user you're logged in as. 🎉
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
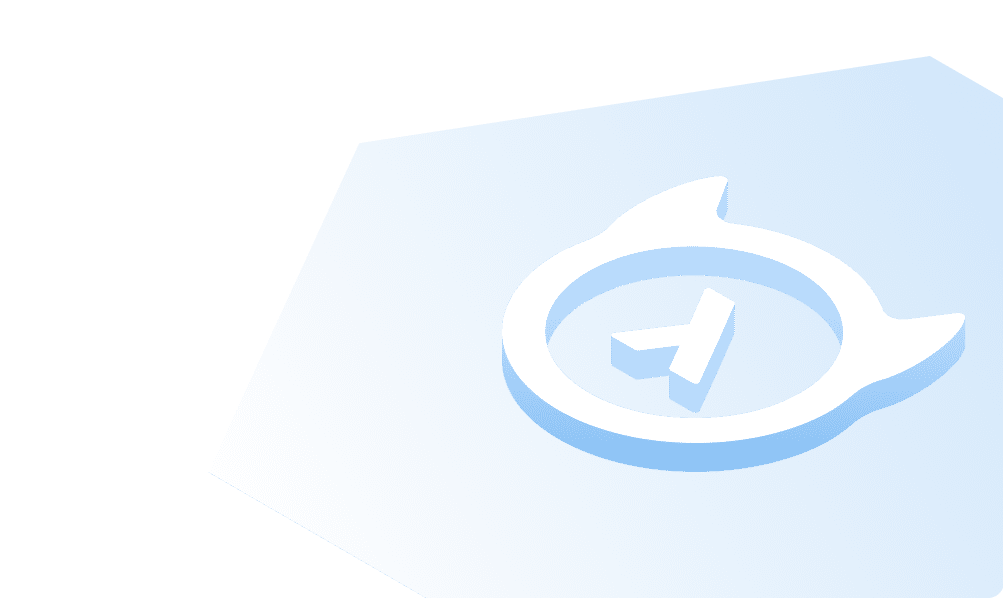
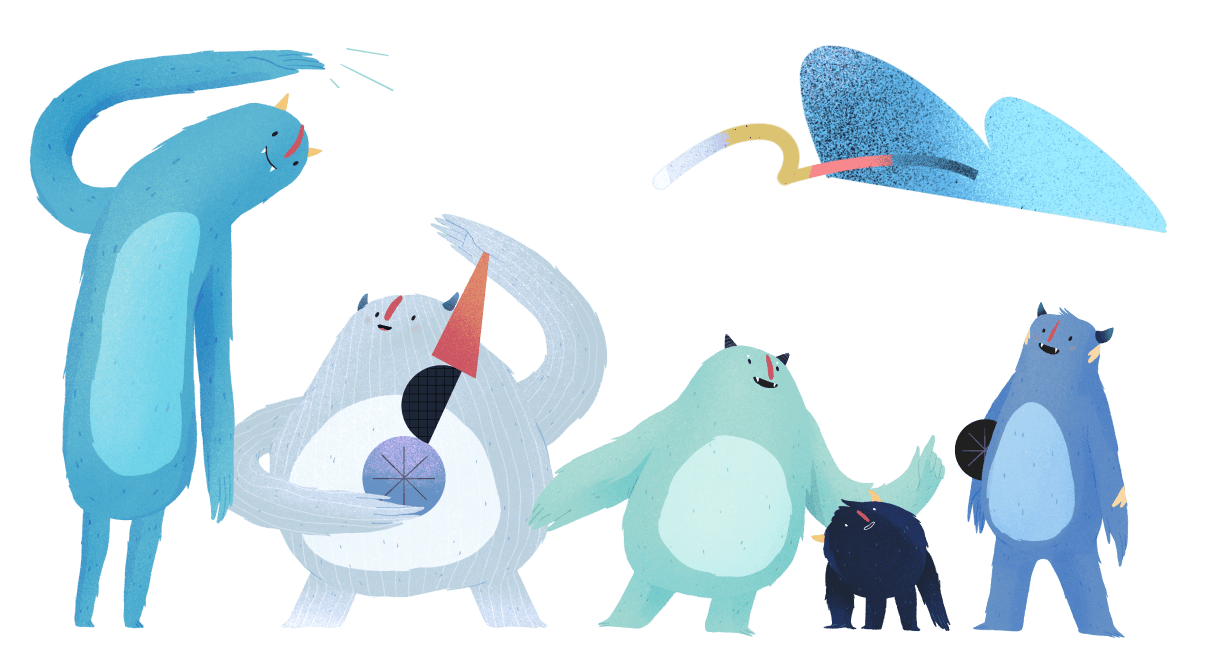