This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Remove todos - Integration
Let us integrate the remove todos feature in our React Native app. Firstly import gql
and define the mutation in src/screens/components/Todo/TodoItem.js
.
+ const REMOVE_TODO = gql`+ mutation ($id: Int) {+ delete_todos (+ where: {+ id: {+ _eq: $id+ }+ }+ ) {+ affected_rows+ }+ }+`;
Firstly use the useMutation
hook with the above mutation to generate the deleteTodo
function.
+ const [deleteTodo, { loading: deleting, error: deleteError }] = useMutation(REMOVE_TODO);
Now, in the TodoItem
component, update the deleteButton
function to use the deleteTodo
function from the useMutation
hook. We also have to update the cache with the todo removal in this case. So we will also write an updateCache
function that we will remove this todo from the UI cache.
+import { FETCH_TODOS } from './Todos';
const deleteButton = () => {if (isPublic) return null;+ const updateCache = (client) => {+ const data = client.readQuery({+ query: FETCH_TODOS,+ variables: {+ isPublic,+ }+ });+ const newData = {+ todos: data.todos.filter((t) => t.id !== item.id)+ }+ client.writeQuery({+ query: FETCH_TODOS,+ variables: {+ isPublic,+ },+ data: newData+ });+ }const remove = () => {if (deleting) return;+ deleteTodo({+ variables: { id: item.id },+ update: updateCache+ });+ };return (<View style={styles.deleteButton}><Iconname="delete"size={26}onPress={remove}+ disabled={deleting}color={"#BC0000"}/></View>);}
This was done similar to the insert_todos
mutation. We have also updated the cache in the update
function. With this, we have a fully functional todo app working :)
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
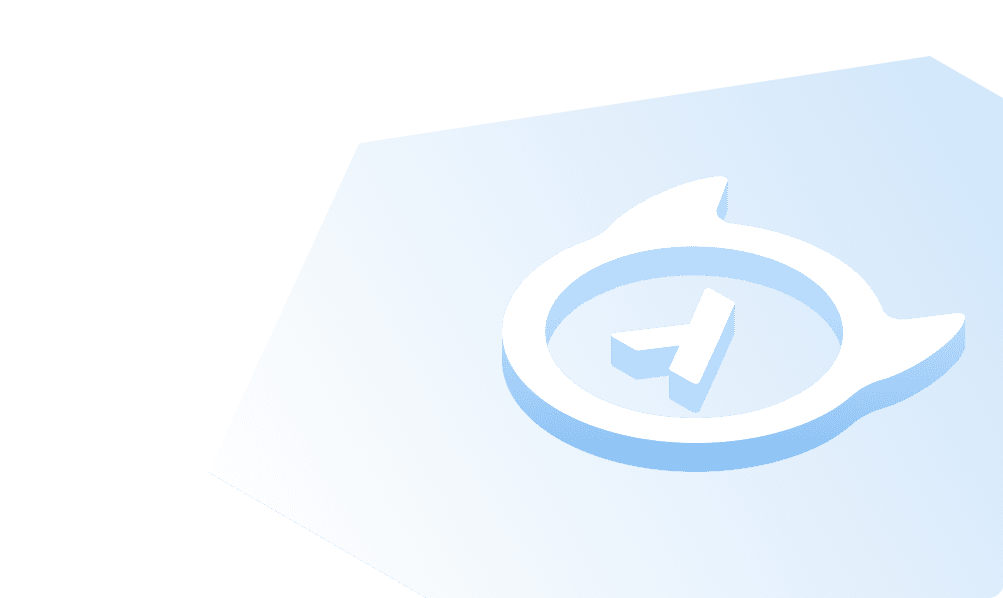
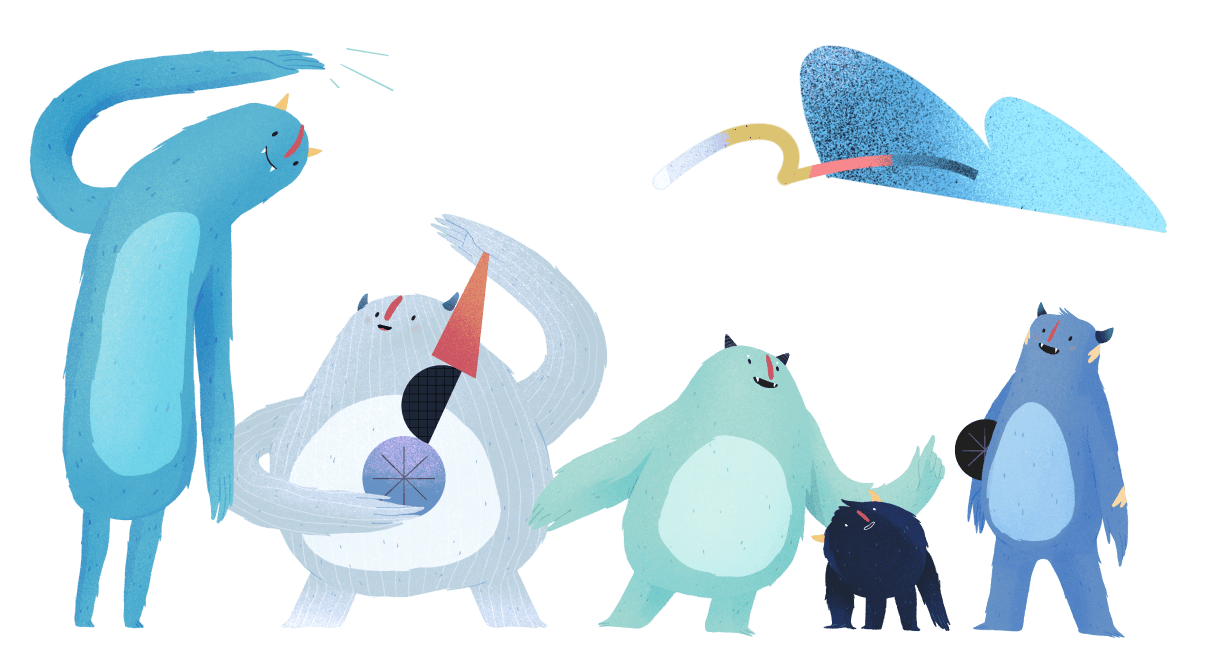