This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Delete mutation and update cache
Now let's do the integration part. Open src/components/Todo/TodoItem.tsx
and add the following code to define the delete mutation
const TOGGLE_TODO = gql`mutation toggleTodo ($id: Int!, $isCompleted: Boolean!) {update_todos(where: {id: {_eq: $id}}, _set: {is_completed: $isCompleted}) {affected_rowsreturning {idtitleis_completed}}}`;+ const REMOVE_TODO = gql`+ mutation removeTodo ($id: Int!) {+ delete_todos(where: {id: {_eq: $id}}) {+ affected_rows+ }+ }+ `;
Let's use this mutation in the hook.
const TodoItem = ({index, todo}: TodoItemType) => {const [todoUpdate] = useMutation(TOGGLE_TODO,...);+ const [todoRemove] = useMutation(REMOVE_TODO);
We have a function defined to handle the button click to remove a todo. Let's update the function to call the todoRemove
function, passing in the right variables.
const removeTodo = (e: React.MouseEvent) => {e.preventDefault();e.stopPropagation();+ todoRemove({+ variables: { id: todo.id },+ });};
This will ensure that the mutation to delete the todo is executed successfully. Now we are still left with updating cache to update the UI.
- const [todoRemove] = useMutation(REMOVE_TODO);+ const [todoRemove] = useMutation(+ REMOVE_TODO,+ {+ update(cache, { data: { delete_todo } }) {+ const existingTodos: any = cache.readQuery({ query: GET_MY_TODOS });+ const newTodos = existingTodos!.todos.filter((t:any) => (t.id !== delete_todo.id));+ cache.writeQuery({+ query: GET_MY_TODOS,+ data: {todos: newTodos}+ });+ }+ }+ );
Try removing a todo now. It should also be removed from the UI once the mutation completes.
Mapping Types
To add the type definitions, let's import the auto-generated RemoveTodoMutationFn
.
- import { GetMyTodosQuery, Todos } from '../../generated/graphql';+ import { GetMyTodosQuery, Todos, RemoveTodoMutationFn } from '../../generated/graphql';
Let's apply this to the relevant useMutation section.
- const [todoRemove] = useMutation(+ const [todoRemove] = useMutation<RemoveTodoMutationFn>(REMOVE_TODO,{update(cache, { data }) {- const existingTodos: any = cache.readQuery({ query: GET_MY_TODOS });+ const existingTodos = cache.readQuery<GetMyTodosQuery>({ query: GET_MY_TODOS });- const newTodos = existingTodos!.todos.filter((t:any) => (t.id !== todo.id));+ const newTodos = existingTodos!.todos.filter(t => (t.id !== todo.id));- cache.writeQuery({+ cache.writeQuery<GetMyTodosQuery>({query: GET_MY_TODOS,data: {todos: newTodos}});}});
That's the end of the delete mutation.
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
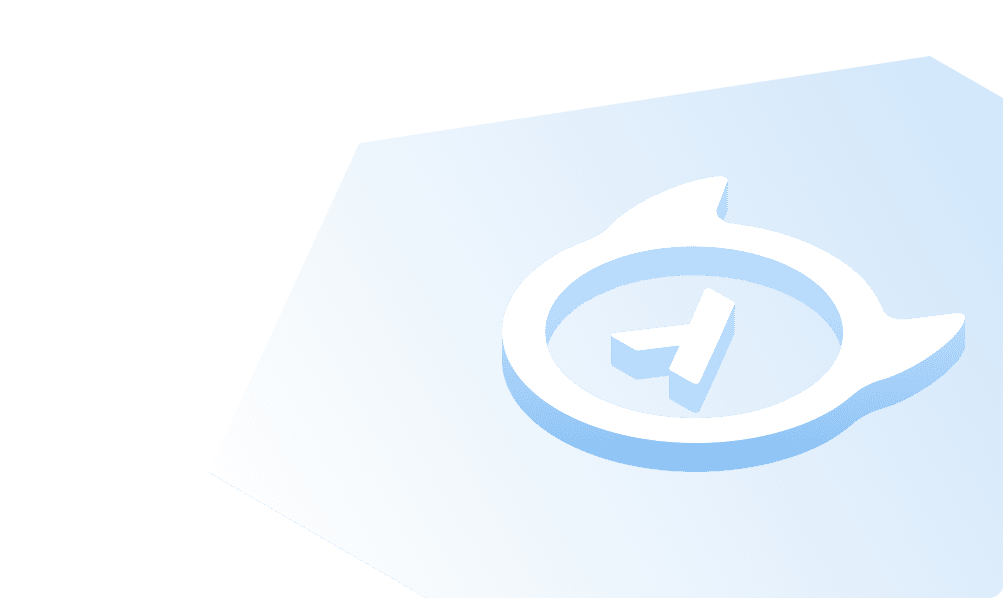
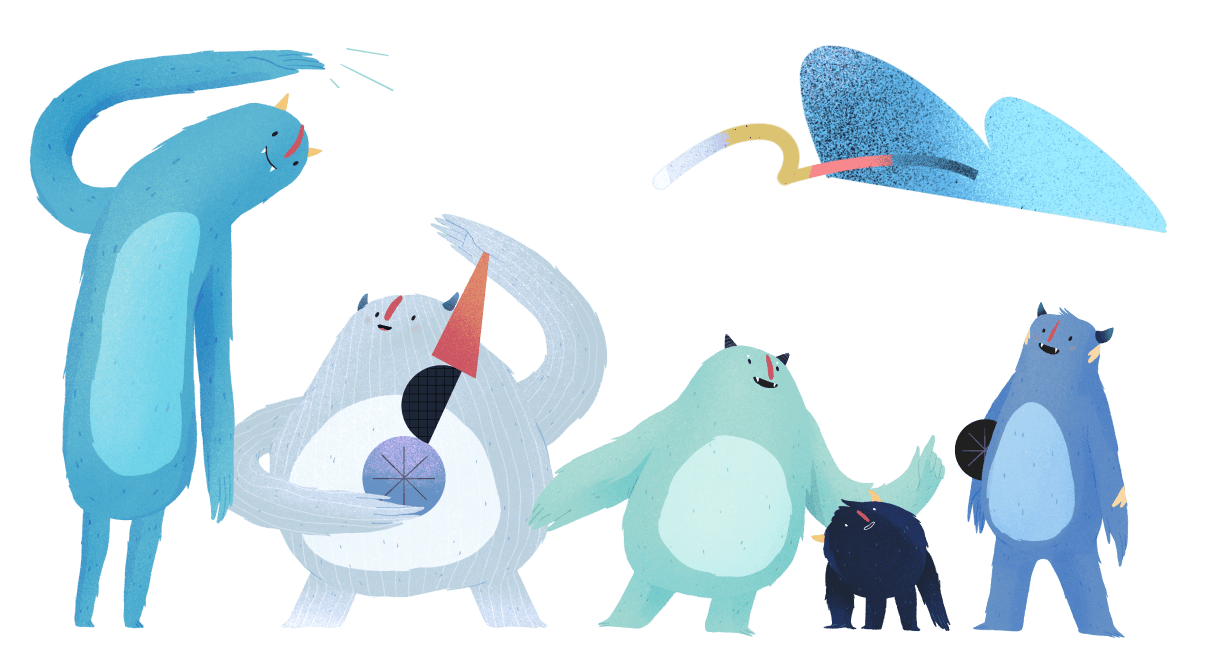