This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Create Subscription and Render Result
Add subscription query
So let's define the graphql subscription to be used.
Open src/components/OnlineUsers.vue
and add the following code, below the other import.
<script>+ import gql from 'graphql-tag'+ const SUBSCRIPTION_ONLINE_USERS = gql`+ subscription getOnlineUsers {+ online_users(order_by: {user: {name: asc }}) {+ id+ user {+ name+ }+ }+ }+ `;
We are defining the graphql subscription query to fetch the online user data. Now let's define a smart subscription.
<script setup lang="ts">import { useMutation, useResult, useSubscription } from "@vue/apollo-composable"const onlineUsersSubscription = useSubscription(SUBSCRIPTION_ONLINE_USERS)- const onlineUsers = [{ user: { name: "someUser1" } }, { user: { name: "someUser2" } }]+ const onlineUsers = useResult(onlineUsersSubscription.result, [], (data) => data.online_users)const UPDATE_LASTSEEN_MUTATION = gql`mutation updateLastSeen($now: timestamptz!) {update_users(where: {}, _set: { last_seen: $now }) {affected_rows}}`const updateLastSeenMutation = useMutation(UPDATE_LASTSEEN_MUTATION, {variables: () => ({now: new Date().toISOString(),}),})setInterval(async () => {try {updateLastSeenMutation.mutate()} catch (e) {console.log(e)}}, 30000)</script>
How does this work?
We are using the useSubscription()
method to define the subscription query, which functions similar to queries. The online_users
prop gives the result of the real-time data for the query we have made.
Refresh your vue app and see yourself online! Don't be surprised ;) There could be other users online as well.
Awesome! You have completed basic implementations of a GraphQL Query, Mutation and Subscription. Easy isn't it?
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
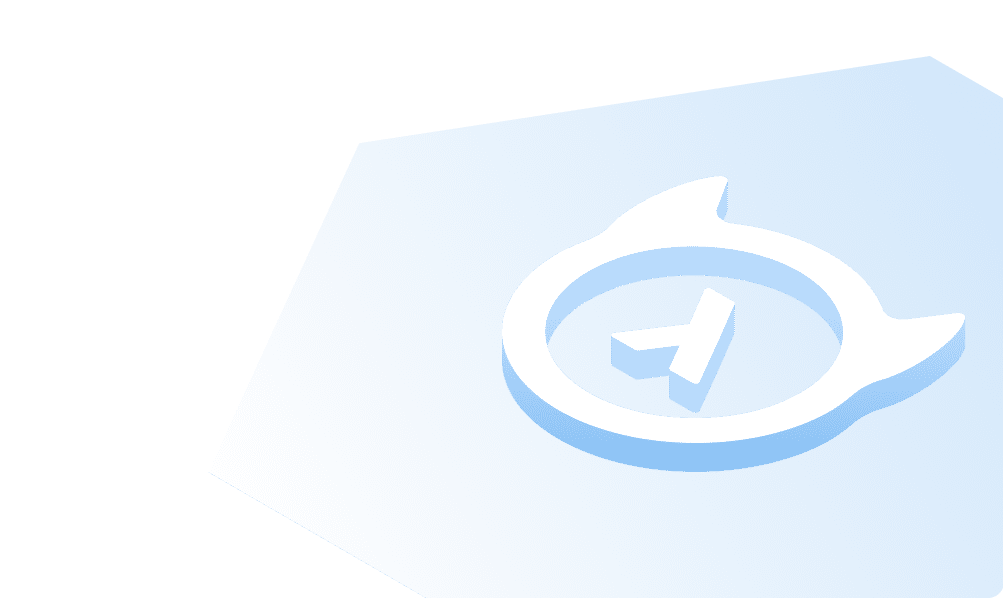
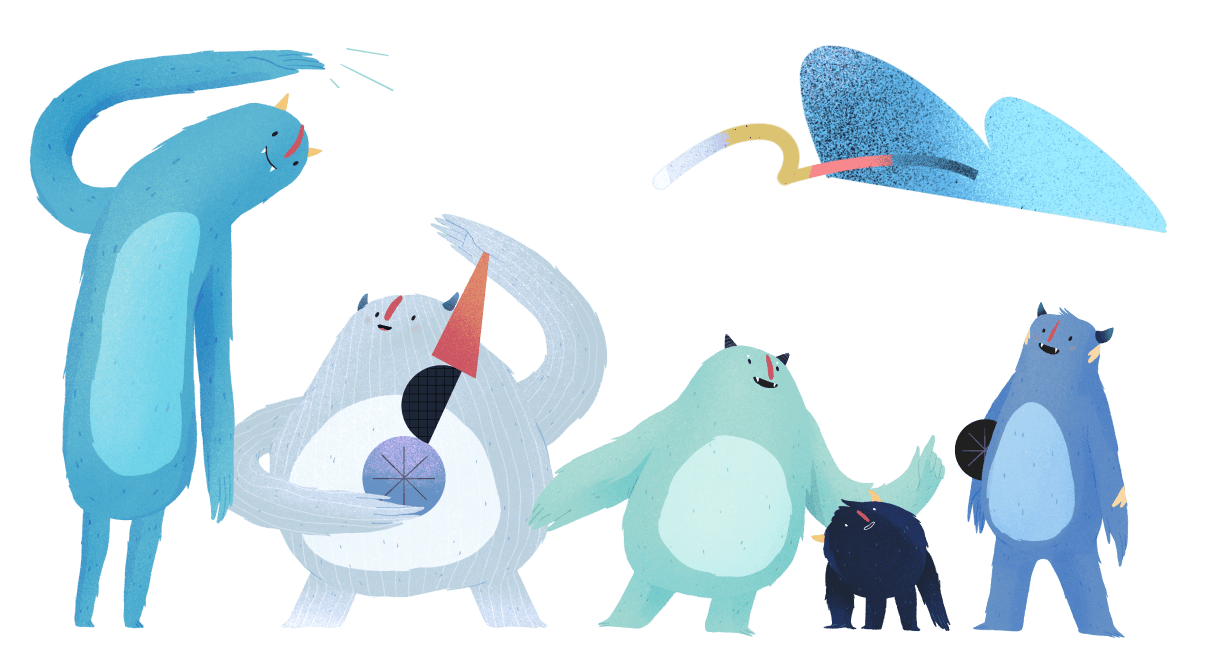