This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Set up a GraphQL client with Apollo
Hope you got the app-boilerplate code up and running. We will use Apollo as GraphQL client in this tutorial.
Apollo gives a neat abstraction layer and an interface to your GraphQL server. You don't need to worry about constructing your queries with request body, headers and options, that you might have done with axios
or fetch
say. You can directly write queries and mutations in GraphQL and they will automatically be sent to your server via your apollo client instance.
React Apollo Installation
Let's get started by installing apollo client & peer graphql dependencies:
$ npm install @apollo/client graphql rescript-apollo-client
What are these dependencies?
@apollo/client
is a GraphQL client librarygraphql
package contains core utilities which are used by other graphql librariesrescript-apollo-client
contains ReScript bindings for the Apollo Client
Let's add rescript-apollo-client
to bs-dependencies
Open bsconfig.json
and update bs-dependencies
{...- "bs-dependencies": ["@rescript/react"]+ "bs-dependencies": ["@rescript/react", "rescript-apollo-client"],}
Create Apollo Client Instance
Let's create a function that creates and return a apollo client instance.
Open src/components/App.res
and add the following code to create apollo client and App component.
let createApolloClient = authToken => {let headers = {"Authorization": `Bearer ${authToken}`,}let httpLink = ApolloClient.Link.HttpLink.make(~uri=_ => "https://hasura.io/learn/graphql",~headers=Obj.magic(headers),(),)let client = {open ApolloClientmake(~cache=Cache.InMemoryCache.make(),~connectToDevTools=true,~defaultOptions=DefaultOptions.make(~mutate=DefaultMutateOptions.make(~awaitRefetchQueries=true, ()),(),),~link=httpLink,(),)}client}
Let's try to understand what is happening here.
HttpLink and InMemoryCache
We are creating an HttpLink
to connect ApolloClient with the GraphQL server. As you know already, our GraphQL server is running at https://hasura.io/learn/graphql
At the end, we instantiate ApolloClient by passing in our HttpLink and a new instance of InMemoryCache
(recommended caching solution). We are wrapping all of this in a function which will return the client.
Next, let's create the apollo client instance inside App
and pass the client prop to <ApolloProvider>
component.
@react.componentlet make = (~idToken) => {let {loading, logout} = useAuth0()if loading {<div> {React.string("Loading...")} </div>} else {+ let client = createApolloClient(idToken)+ <ApolloClient.React.ApolloProvider client={client}><div></div>+ </ApolloClient.React.ApolloProvider>}}
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
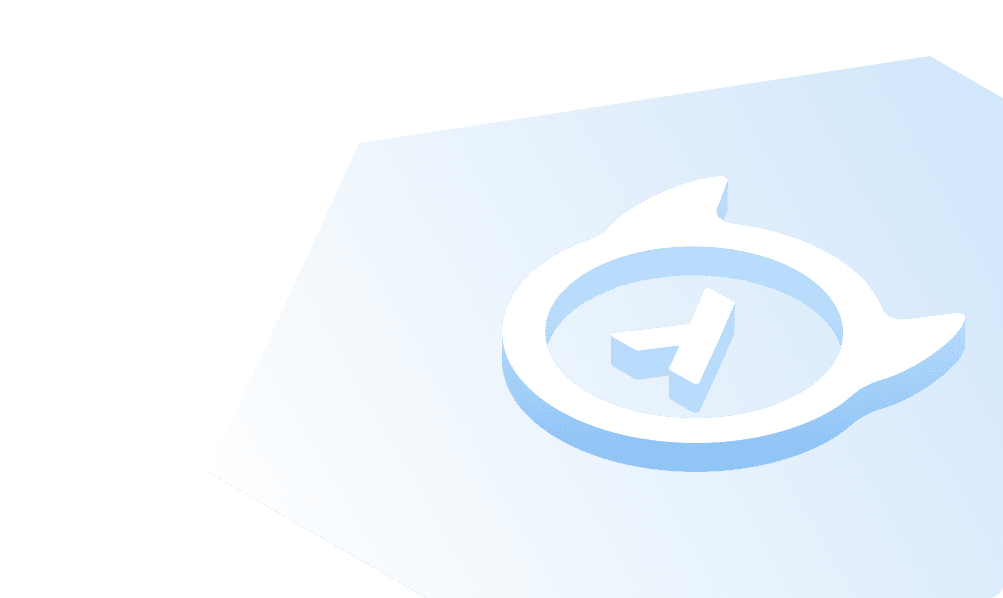
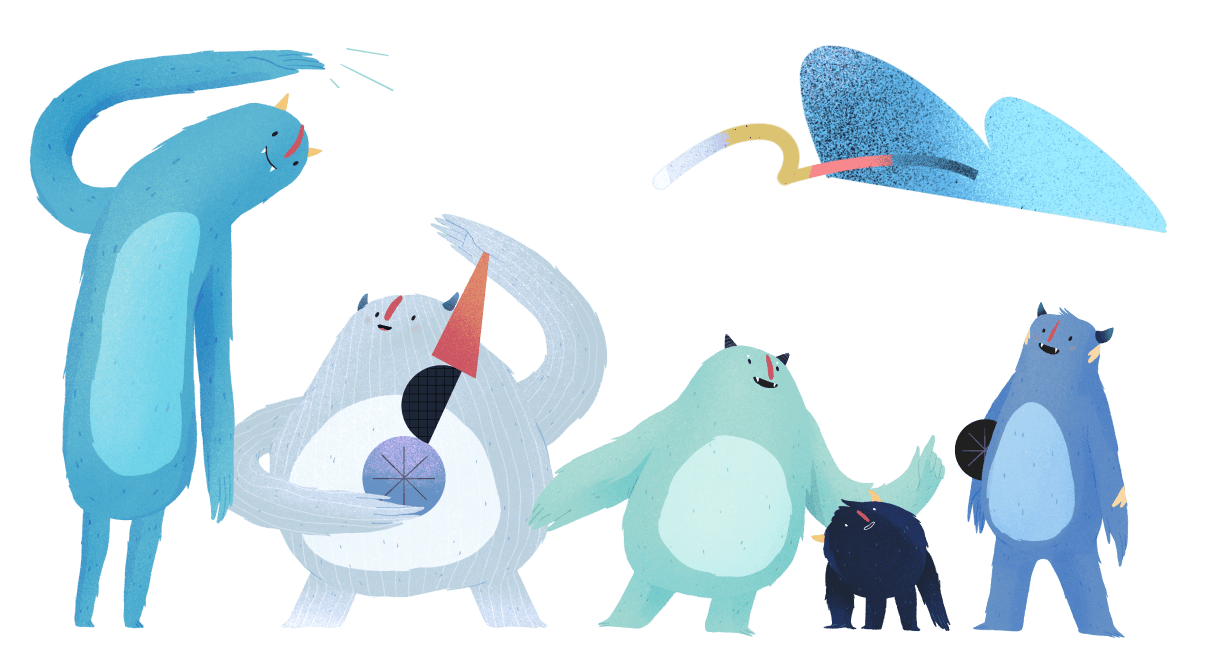