This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Mutation and update cache
Now let's do the integration part. Open src/components/Todo/TodoItem.res
and add the following code to define the delete mutation
module RemoveTodoMutation = %graphql(`mutation removeTodo($id: Int!) {delete_todos(where: { id: { _eq: $id } }) {affected_rows}}`)
We have a function defined to handle the button click to remove a todo. Let's update the function to use removeTodoMutation
mutate function.
let removeTodo = e => {ReactEvent.Mouse.preventDefault(e)ReactEvent.Mouse.stopPropagation(e)removeTodoMutate(~update=({readQuery, writeQuery}, {data: _data}) => {let existingTodos = readQuery(~query=module(TodosQuery), ())switch existingTodos {| Some(todosResult) =>switch todosResult {| Ok({todos}) => {let newTodos = Js.Array2.filter(todos, t => t.id !== todo.id)let _ = writeQuery(~query=module(TodosQuery), ~data={todos: newTodos}, ())}| _ => ()}| None => ()}}, {id: todo.id})->ignore}
Let's pass optimistic response for instant UI update.
let removeTodo = e => {ReactEvent.Mouse.preventDefault(e)ReactEvent.Mouse.stopPropagation(e)removeTodoMutate(+ ~optimisticResponse=_variables => {+ delete_todos: Some(+ (+ {+ affected_rows: 1,+ __typename: "todos_mutation_response",+ }: RemoveTodoMutation.RemoveTodoMutation_inner.t_delete_todos+ ),+ ),+ },~update=({readQuery, writeQuery}, {data: _data}) => {let existingTodos = readQuery(~query=module(TodosQuery), ())switch existingTodos {| Some(todosResult) =>switch todosResult {| Ok({todos}) => {let newTodos = Js.Array2.filter(todos, t => t.id !== todo.id)let _ = writeQuery(~query=module(TodosQuery), ~data={todos: newTodos}, ())}| _ => ()}| None => ()}},{id: todo.id},)->ignore}
Did you find this page helpful?
Start with GraphQL on Hasura for Free
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
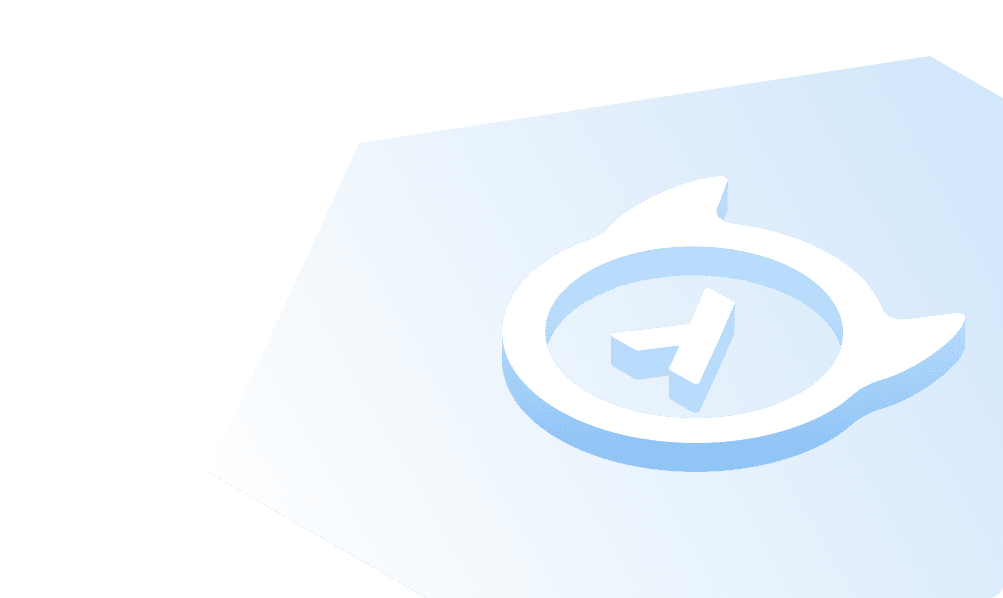
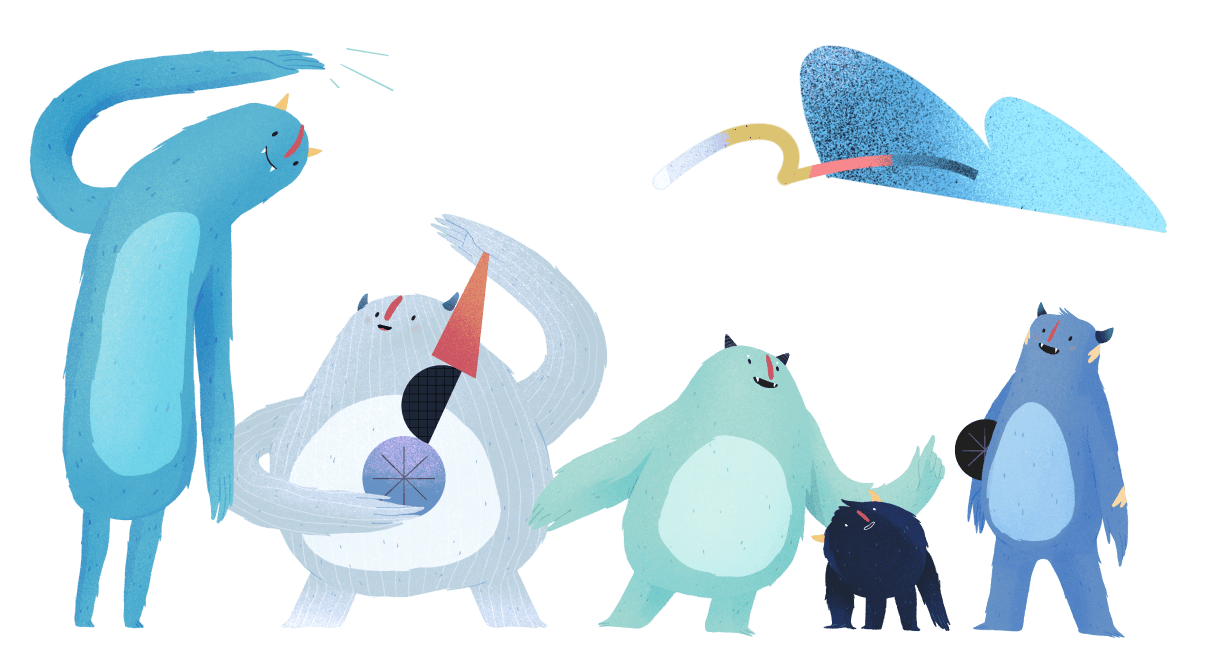