useQuery hook
In this section, we will implement GraphQL Queries and integrate with the react UI.
Create a new file with name TodosQuery.res and add the following code to create a todos query ReScript module.
let make = %graphql(`query {todos(where: { is_public: { _eq: false } }order_by: [{ created_at: desc }]) {idtitlecreated_atis_completed}}`)
%graphql
is a GraphQL primitive from graphql-ppx. In addition to creating a query that you can pass to the client, it will also generate the types of the data that we get back from the GraphQL server.
We created a separate module for this query to reuse it in multiple places.
We can intergate the GraphQL query in a React component as follows
@react.componentlet make = () => {let todosResult = TodosQuery.use()switch todosResult {| {data: Some({todos})} => <TodoPrivateList todos={todos} />| _ => React.null}}
How does this work?
TodosQuery.use()
uses useQuery
React hook from Apollo and fetches the data automatically.
When you use the useQuery
React hook, Apollo returns the data along with other properties. Most important ones are:
loading
: A boolean that indicates whether the request is in flight. If loading is true, then the request hasn't finished. Typically this information can be used to display a loading spinner.
error
: A runtime error with graphQLErrors and networkError properties. Contains information about what went wrong with your query.
data
: An object containing the result of your GraphQL query. This will contain our actual data from the server. In our case, it will be the todo data.
You can read more about other properties that result object contains here
Using the data
property, we are parsing the results from the server. In our query, data
property has an array todos
which can be mapped over to render each TodoItem
. We can use ReScript's Pattern Matching feature to destructure todos from todosResult.
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
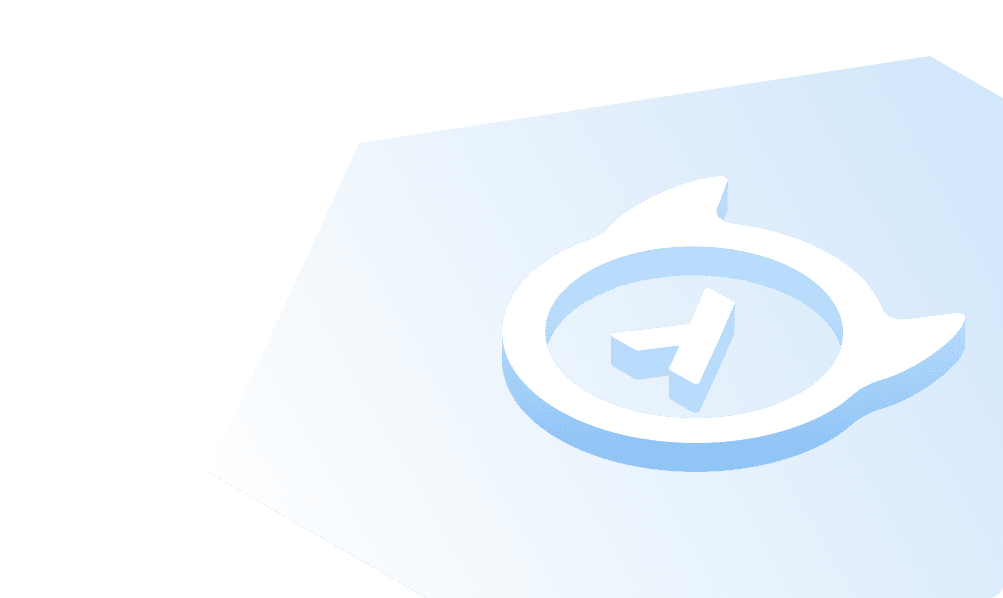
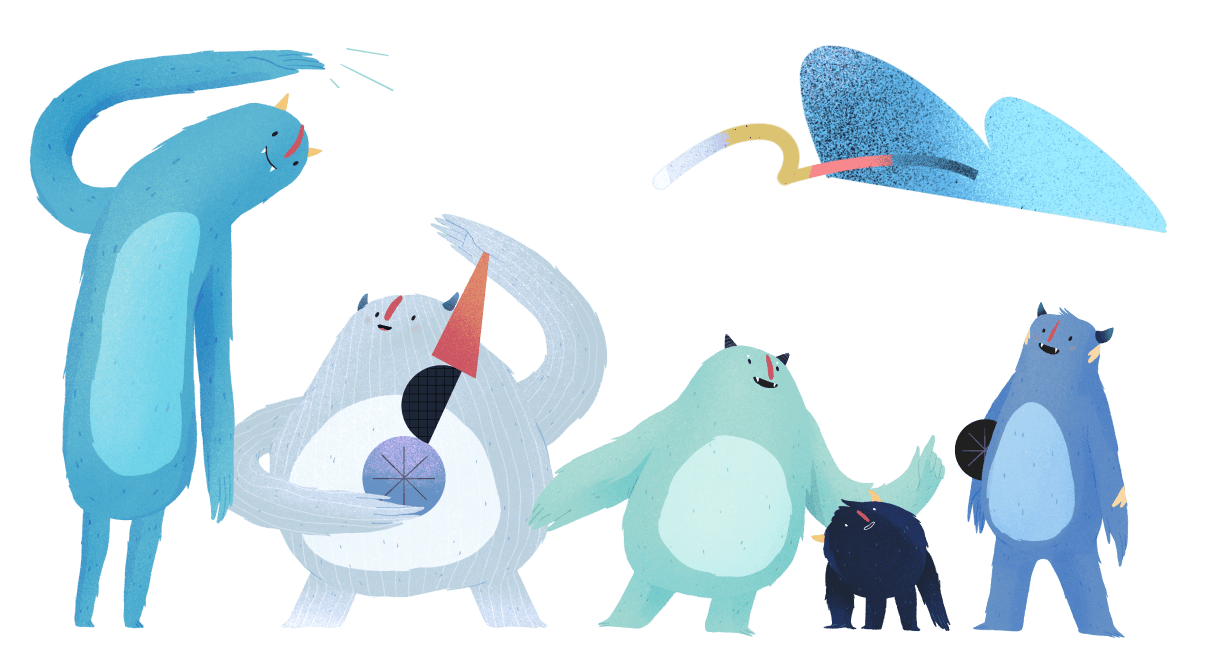