This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Create Subscription and Render Result
Let's create a ReScript module for online users subscription query
module OnlineUsersSubscription = %graphql(`subscription getOnlineUsers {online_users(order_by: [{ user: { name: asc } }]) {iduser {name}}}`)
We can integrate the subscription query in react query as shown below.
@react.componentlet make = () => {let onlineUsersResult = OnlineUsersSubscription.use()switch onlineUsersResult {| {loading: true} => <span> {React.string("Loading...")} </span>| {data: Some({online_users})} => <OnlineUsers online_users={online_users} />| {error: Some(_error)} => <span> {React.string("Error!")} </span>}}
How does this work?
Since OnlineUsersSubscription
is a GraphQL subscription query, OnlineUsersSubscription.use()
uses useSubscription
React hook of Apollo. We are using the useSubscription
React hook which returns properties (similar to useQuery
and useMutation
React hooks). The data
property gives the result of the realtime data for the query we have made.
Refresh your react app and see yourself online! Don't be surprised; There could be other users online as well.
Awesome! You have completed implementations of a GraphQL Query, Mutation and Subscriptions.
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
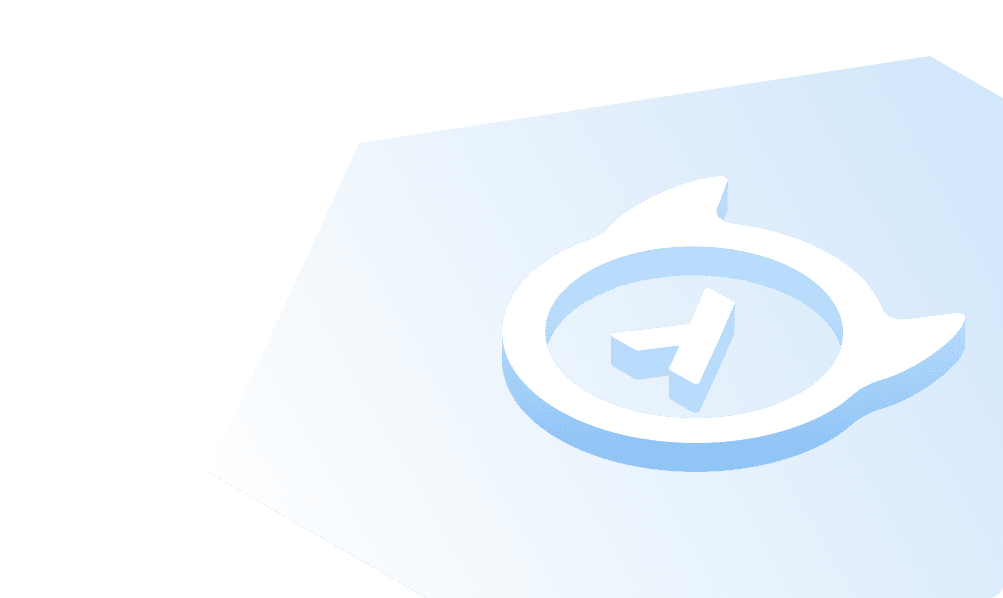
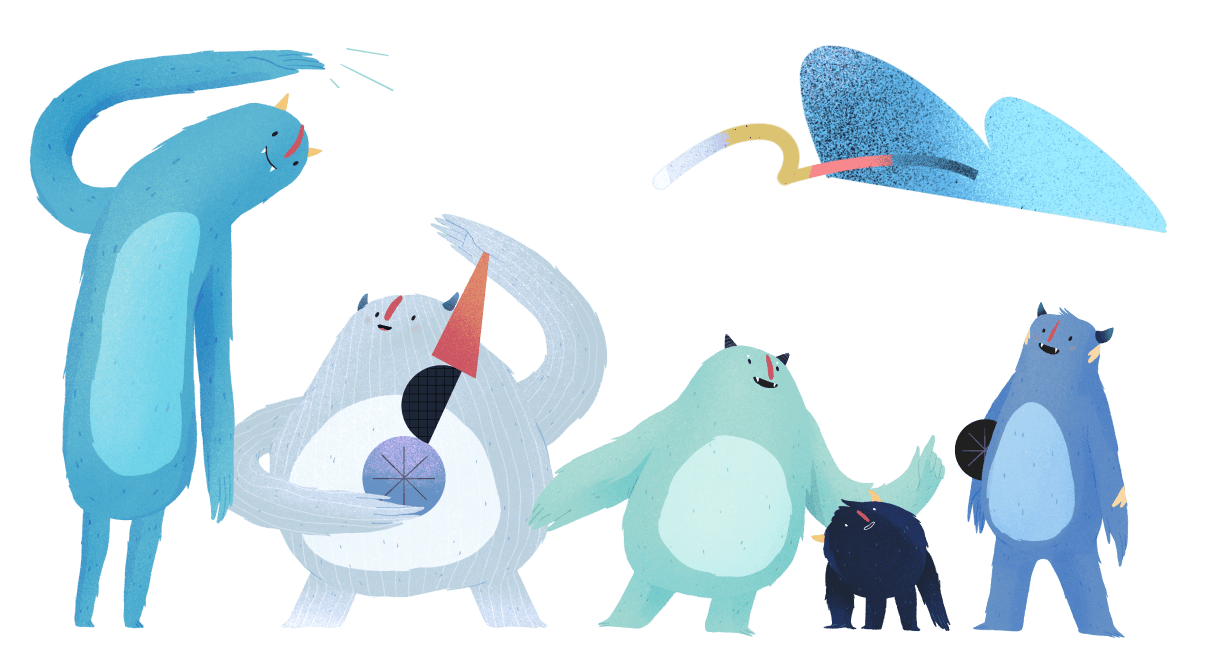