This course is no longer maintained and may be out-of-date. While it remains available for reference, its content may not reflect the latest updates, best practices, or supported features.
Subscriptions to show online users
We cruised through our GraphQL queries and mutations. We queried for todos, added a new todo, updated an existing todo, removed an existing todo.
Now let's get to the exciting part.
GraphQL Subscriptions
We have a section of UI which displays the list of online users. So far we have made queries to fetch data and display them on the UI. But typically online users data is dynamic.
We can make use of GraphQL Subscription API to get realtime data from the graphql server to efficiently handle this.
But but but...
We need to tell the server that the user who is logged in is online. We have to poll our server to do a mutation which updates the last_seen
timestamp value of the user.
We have to make this change to see yourself online first. Remember that you are already logged in, registered your data in the server, but not updated your last_seen
value.?
The goal is to update every few seconds from the client that you are online. Ideally you should do this after you have successfully authenticated with Auth0. So let's update some code to handle this.
Open components/OnlineUsers/OnlineUsersWrapper.js
and add the following imports
- import React from "react";+ import React, { useEffect, useState } from "react";+ import { useMutation, gql } from "@apollo/client";
In useEffect
, we will create a setInterval
to update the last_seen of the user every 30 seconds.
const OnlineUsersWrapper = () => {+ const [onlineIndicator, setOnlineIndicator] = useState(0);+ let onlineUsersList;+ useEffect(() => {+ // Every 20s, run a mutation to tell the backend that you're online+ updateLastSeen();+ setOnlineIndicator(setInterval(() => updateLastSeen(), 20000));++ return () => {+ // Clean up+ clearInterval(onlineIndicator);+ };+ }, []);
Now let's write the definition of the updateLastSeen
.
const OnlineUsersWrapper = () => {const [onlineIndicator, setOnlineIndicator] = useState(0);let onlineUsersList;useEffect(() => {// Every 20s, run a mutation to tell the backend that you're onlineupdateLastSeen();setOnlineIndicator(setInterval(() => updateLastSeen(), 20000));return () => {// Clean upclearInterval(onlineIndicator);};}, []);+ const UPDATE_LASTSEEN_MUTATION = gql`+ mutation updateLastSeen($now: timestamptz!) {+ update_users(where: {}, _set: { last_seen: $now }) {+ affected_rows+ }+ }+ `;+ const [updateLastSeenMutation] = useMutation(UPDATE_LASTSEEN_MUTATION);+ const updateLastSeen = () => {+ // Use the apollo client to run a mutation to update the last_seen value+ updateLastSeenMutation({+ variables: { now: new Date().toISOString() }+ });+ };
Again, we are making use of useMutation
React hook to update the users
table of the database.
Great! Now the metadata about whether the user is online will be available in the backend. Let's now do the integration to display realtime data of online users.
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
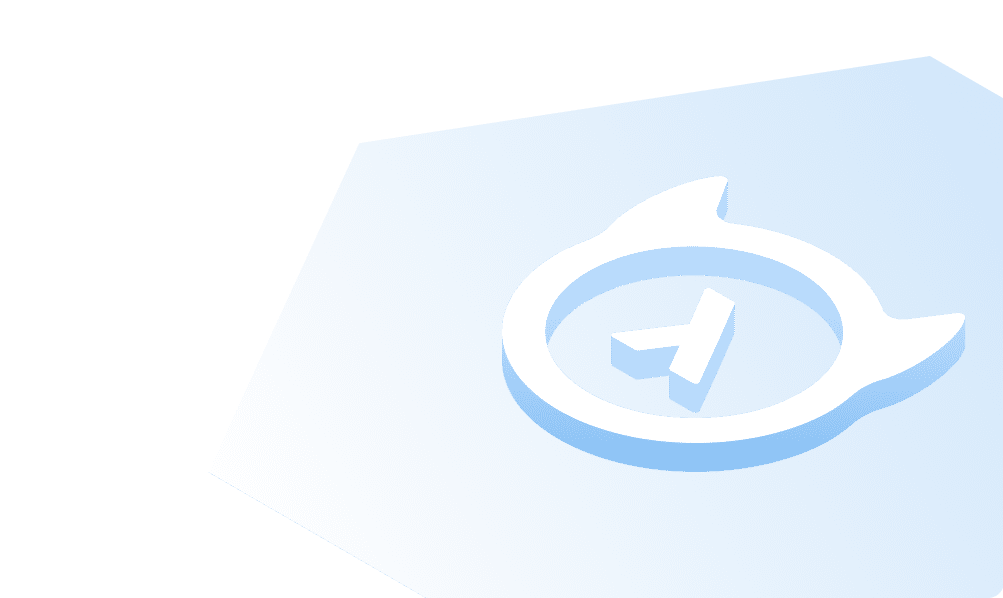
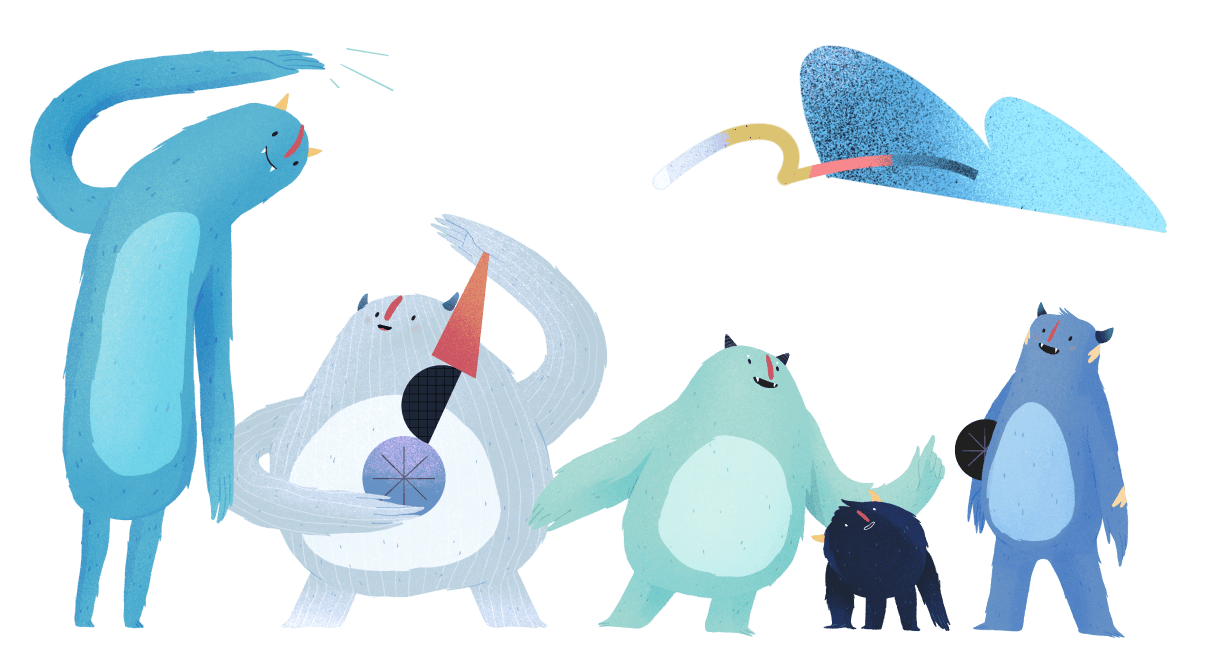