Getting started with React Query and GraphQL
Updating our base example
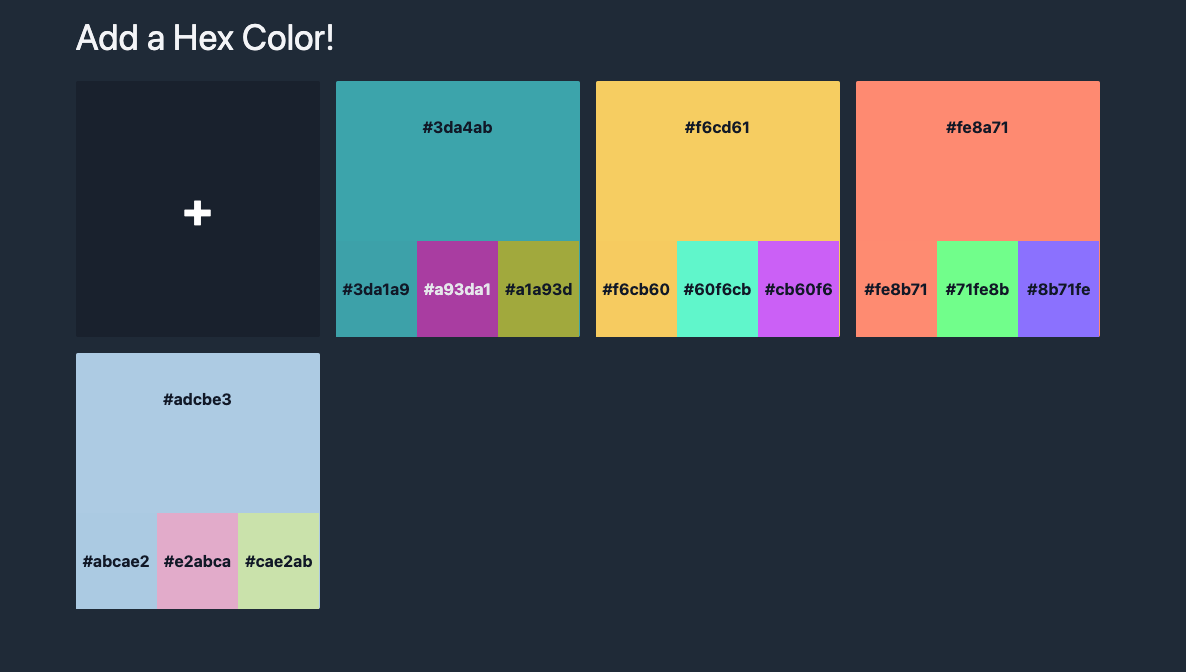
Invalidating Cache vs Subscription
Invalidating Cache
//Dependency
import { useMutation, useQueryClient } from "react-query";
// Client Reference
const queryClient = useQueryClient();
// Mutation Hook
const { isLoading, isError, isSuccess, data, mutate } = useMutation(
(hex) =>
fetch("https://intent-shad-91.hasura.app/v1/graphql", {
method: "POST",
body: JSON.stringify({
query: `
mutation InsertColorOne( $hex: String ){
insert_color_one(object: {color: $hex}) {
color
complementary_colors {
color
}
}
}
`,
variables: {
hex,
},
}),
})
.then((res) => res.json())
.then((res) => res.data),
{
onSuccess: ({insert_color_one}) => {
queryClient.setQueryData(["data"], ({color}) => {
return { color: [...color, insert_color_one] };
});
},
}
);
Creating Subscriptions
import { useEffect, useState } from "react";
import { useQueryClient } from "react-query";
const url = "ws://intent-shad-91.hasura.app/v1/graphql";
export const useColorSubscription = () => {
const queryClient = useQueryClient();
const [isSubscribing, setIsSubscribing] = useState(false);
const [isSubscribingSuccess, setIsSubscribingSuccess] = useState(false);
useEffect(() => {
const ws = new WebSocket(url, "graphql-ws");
setIsSubscribing(true);
ws.onopen = () => {
ws.send(JSON.stringify({ type: "connection_init", payload: {} }));
ws.send(
JSON.stringify({
id: "1",
type: "start",
payload: {
// variables: {},
extensions: {},
operationName: "GetColors",
query: `subscription GetColors {
color {
color
complementary_colors {
color
}
}
}`,
},
})
);
};
ws.onmessage = (event) => {
const msg = JSON.parse(event.data);
if (msg.type == "data") {
setIsSubscribingSuccess(true);
setIsSubscribing(false);
const data = msg.payload.data.color;
queryClient.setQueriesData("colors", data);
}
};
return () => {
ws.send(JSON.stringify({ id: "1", type: "stop" }));
ws.close();
};
}, []);
return { isSubscribing, isSubscribingSuccess };
};
const { data } = useQuery("colors", () => [], {
refetchOnMount: false,
refetchOnWindowFocus: false,
refetchOnReconnect: false,
refetchIntervalInBackground: false,
});
const { isSubscribing, isSubscribingSuccess } = useColorSubscription();
{isSubscribing && <p className="text-2xl text-gray-200">Loading...</p>}
{isSubscribingSuccess &&
data.map((item, key) => {
return ({/* My Code */})
}
</div>
);
})}
const { isLoading, isError, isSuccess, data, mutate } = useMutation((hex) =>
fetch("https://intent-shad-91.hasura.app/v1/graphql", {
method: "POST",
body: JSON.stringify({
query: `
mutation InsertColorOne( $hex: String ){
insert_color_one(object: {color: $hex}) {
color
complementary_colors {
color
}
}
}
`,
variables: {
hex,
},
}),
})
.then((res) => res.json())
.then((res) => res.data)
);
Implementing GraphQL Codegen
mutation InsertColorOne($hex: String) {
insert_color_one(object: { color: $hex }) {
color
complementary_colors {
color
}
}
}
// Dependencies for generator script
yarn add graphql
yarn add -D @graphql-codegen/cli
// Init Script
yarn graphql-codegen init
overwrite: true
schema: "https://intent-shad-91.hasura.app/v1/graphql"
documents: "./src/queries/**/**.gql"
generates:
src/generated/graphql.ts:
plugins:
- "typescript"
- "typescript-operations"
- "typescript-react-query"
config:
fetcher:
endpoint: "https://intent-shad-91.hasura.app/v1/graphql"
./graphql.schema.json:
plugins:
- "introspection"
// Imports
import { useInsertColorOneMutation } from "./generated/graphql";
// Instantiate
const { mutate, isLoading, isSuccess } = useInsertColorOneMutation();
// Use
() => {mutate({ hex })}
Related reading