Getting Started with Hot Chocolate .NET GraphQL Server
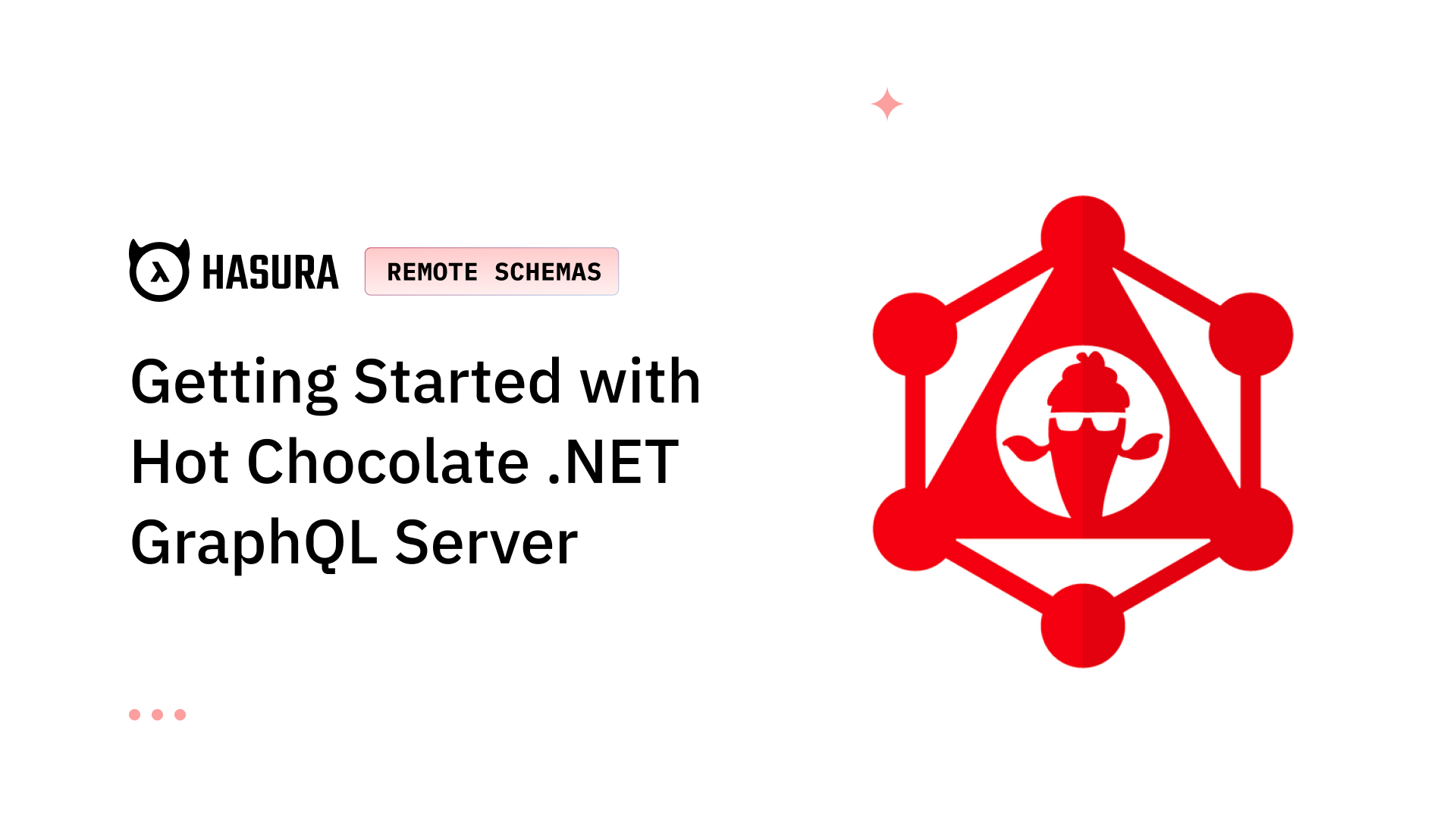
- .NET Core SDK
- Any code editor of your choice (we recommend Visual Studio Code)
Step 1: Create a New .NET Core Web API Project
dotnet new webapi --no-https
Step 2: Install Hot Chocolate GraphQL in the .NET Core Project
dotnet add package HotChocolate.AspNetCore
dotnet add package HotChocolate.Data
Step 3: Create Your GraphQL Schema
- Create a folder called Models at the root of your project and create the Actor.cs file inside the folder with the following code snippet
//Actor.cs
namespace Models
{
public class Actor
{
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
- Create another C# file called Movie.cs inside the Models folder and add the following code snippet
//Movie.cs
namespace Models
{
public class Movie
{
public int Id { get; set; }
public string Title { get; set; }
public List<Actor> Actors { get; set; }
}
}
Step 4: Create Your Dataset
- Create a folder called Data at the root of your project, create the SeedData.cs file inside the folder, and add the following code snippet
//SeedData.cs
using Models;
namespace Data
{
public class Seed
{
public static List<Movie> SeedData()
{
var actors = new List<Actor>
{
new Actor
{
FirstName = "Bob",
LastName = "Kante"
},
new Actor
{
FirstName = "Mary",
LastName = "Poppins"
}
};
var movies = new List<Movie>
{
new Movie
{
Id = 1,
Title = "The Rise of the GraphQL Warrior",
Actors = actors
},
new Movie
{
Id = 2,
Title = "The Rise of the GraphQL Warrior Part 2",
Actors = actors
}
};
return movies;
}
}
}
Step 5: Define Your Data Resolvers
- At the root of your project, create a folder called Resolvers
- Inside the Resolvers folder, create a C# file called Query.cs and paste the following code snippet
using Data;
using Models;
namespace Resolvers
{
public class Query
{
public List<Movie> GetMovies() =>
Seed.SeedData();
public Movie GetMovieById(int id) =>
Seed.SeedData().FirstOrDefault(x => x.Id == id);
}
}
Step 6: Configure Your GraphQL Server
//Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services
.AddGraphQLServer()
.AddQueryType<Query>();
}
//Startup.cs
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app
.UseRouting()
.UseEndpoints(endpoints =>
{
endpoints.MapGraphQL();
});
}
Step 7: Execute Your GraphQL Query
- Start the GraphQL server with the following command
dotnet run
- Open your browser and head over to the port your GraphQL server is listening on (ours is http://localhost:5000/graphql) to open the Hot Chocolate built-in GraphQL IDE Banana Cake Pop
- Copy the following code snippet into the operations window and click on the Run button to test the GraphQL API
query {
movies {
title
actors {
firstName
}
}
}
Step 8: Create A Public Endpoint For Your GraphQL API
- Download the current version of ngrok
- Register and get a token
- Open your terminal and set the ngrok token with this command: ngrok authtoken YOUR_AUTHTOKEN
- Create a tunnel with this command: ngrok http -host-header=localhost http://localhost:5000
- The output of the last command will list a public forwarding URL, which will point to your local server
Step 9: Create Your Database on Hasura Cloud
- Sign in to Hasura Cloud
- Create a project and click on the Launch Console button
- Navigate to the Data tab and create a new Heroku Postgres database
- After the database has been created, click on the Create Table button in the Data tab and create the table Movies with the following columns as shown below
- Remember to select Id as the Primary key
Step 10: Attach Your Hot Chocolate GraphQL Server With Hasura’s Remote Schema
- Navigate to the Remote Schemas tab in the Hasura Console to add the Hot Chocolate GraphQL server to Hasura's GraphQL schema, as shown below
Step 11: Merge data with Hasura Remote Join
- Click on the Data tab, select the table Movies and head to the Relationships tab to configure a new Remote Schema Relationship as shown below:
Step 12: Test the GraphQL API
- Navigate to the API tab and examine the query explorer. We can see it contains both the schema in the Hot-Chocolate GraphQL server and the automatically generated schema from the Postgres Hasura database instance
- Paste the following query in the query box. (You can also explore the complete GraphQL schema in the sidebar and toggle based on the fields you want)
query MyQuery {
Movies {
Id
MovieUrl
ReleaseDate
movie_metadata {
title
actors {
firstName
}
}
}
}
- Click on the Run button and review the response
Learn More About Hot Chocolate GraphQL and Hasura
Check out the .NET (dotnet) backend course. In this course, you will learn how to integrate .NET (dotnet) in a GraphQL backend server stack with Hasura.
Related reading