Postgres Types Mapped to JavaScript via GraphQL with Hasura
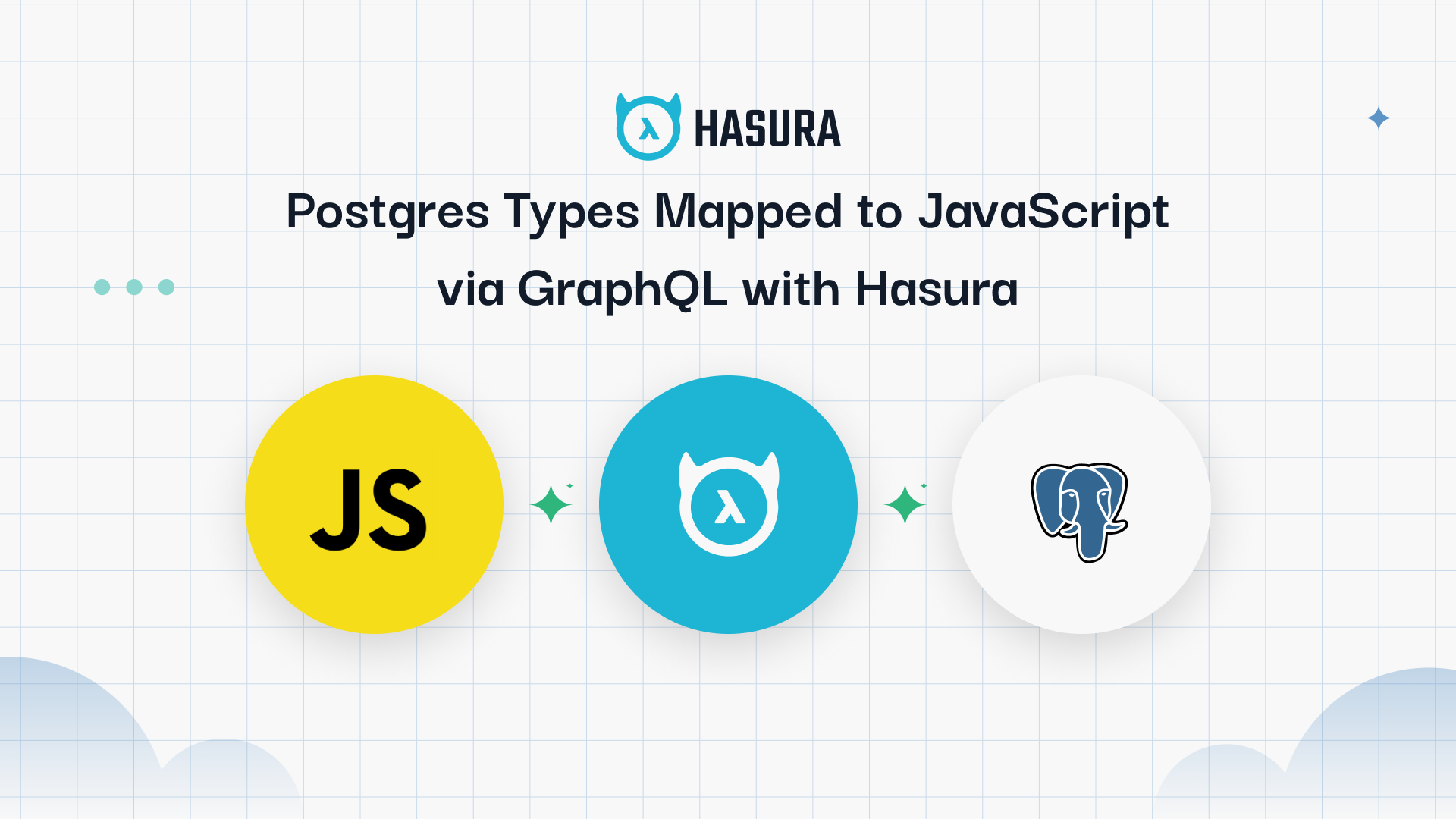
Primary Keys
Primary Key: A primary key in a relational database uniquely identifies the record among many records stored in a table.
- Super Key - A super key is a single or multiple keys that make up a group of keys which identifies a row within a table.
- Candidate Key - A candidate key are attributes that uniquely identify rows in a table. A candidate key is a super key with none of the attributes repeated, thus a natural key within the existing stored data.
- Alternate Key - An alternate key is a column or group of columns that uniquely identify every row in the table. It is a key that isn't particularly selected as a primary key, but can be used as one if the primary key weren't available for some reason.
- Compound Key - A compound key has two or more columns included as the primary key of a table to uniquely identify each row of data.
- Composite Key - An artificial key (think of a generated autonumber, short ID, CUID, or UUID) that uniquely idenfies each record. Also referred to as a surrogate key, these keys are used when a natural key doesn't exist in the schema of the existing table.
- Surrogate Key - See Composite Key.
CREATE OR REPLACE FUNCTION gen_unique_short_id() returns text
language plpgsql
as $$
DECLARE
id text;
BEGIN
id := encode(gen_random_bytes(6), 'base64');
id := replace(id, '/', '_');
id := replace(id, '+', '_');
RETURN id;
END;
$$;
alter function gen_unique_short_id() owner to postgres;
Character Data & Numeric Data Types
Name | Aliases | Description | Hasura Type |
---|---|---|---|
bigint | int8 | signed eight-byte integer | String |
bigserial | serial8 | autoincrementing eight-byte integer | String |
bit [ (n) ] | fixed-length bit string | Implicit | |
bit varying [ (n) ] | varbit [ (n) ] | variable-length bit string | Implicit |
boolean | bool | logical Boolean (true/false) | Bool |
box | rectangular box on a plane | Implicit | |
bytea | binary data (“byte array”) | Implicit | |
character [ (n) ] | char [ (n) ] | fixed-length character string | Char |
character varying [ (n) ] | varchar [ (n) ] | variable-length character string | String |
cidr | IPv4 or IPv6 network address | Implicit | |
circle | circle on a plane | Implicit | |
date | calendar date (year | month | day) | Date | |
double precision | float8 | double precision floating-point number (8 bytes) | Float |
inet | IPv4 or IPv6 host address | Implicit | |
integer | int | int4 | signed four-byte integer | Int |
interval [ fields ] [ (p) ] | time span | Implicit | |
json | textual JSON data | JSON | |
jsonb | binary JSON data | decomposed | JSONB | |
line | infinite line on a plane | Implicit | |
lseg | line segment on a plane | Implicit | |
ltree | labels of data stored in a hierarchical tree-like structure | Implicit | |
geometry | PostGIS Geometry type | Geometry | |
geography | PostGIS Geography type | Geography | |
macaddr | MAC (Media Access Control) address | Implicit | |
macaddr8 | MAC (Media Access Control) address (EUI-64 format) | Implicit | |
money | currency amount | Implicit | |
numeric [ (p | s) ] | decimal [ (p | s) ] | exact numeric of selectable precision | Numeric |
path | geometric path on a plane | Implicit | |
pg_lsn | PostgreSQL Log Sequence Number | Implicit | |
point | geometric point on a plane | Implicit | |
polygon | closed geometric path on a plane | Implicit | |
real | float4 | single precision floating-point number (4 bytes) | Float |
smallint | int2 | signed two-byte integer | Int |
smallserial | serial2 | autoincrementing two-byte integer | Int |
serial | serial4 | autoincrementing four-byte integer | Int |
text | variable-length character string | String | |
time [ (p) ] (without time zone) | time of day (no time zone) | Implicit | |
time [ (p) ] (with time zone) | timetz | time of day | including time zone | Timetz |
timestamp [ (p) ] (without time zone) | date and time (no time zone) | Implicit | |
timestamp [ (p) ] (with time zone) | timestamptz | date and time | including time zone | Timestamptz |
tsquery | text search query | Implicit | |
tsvector | text search document | Implicit | |
txid_snapshot | user-level transaction ID snapshot | Implicit | |
uuid | universally unique identifier | Implicit | |
xml | XML data | Implicit |
Related reading