Write event webhook
Now let's move to the second use-case of sending an email when a user registers on the app.
When the user registers on the app using Auth0, we insert a new row into the users
table to keep the user data in sync. Remember the Auth0 rule we wrote during signup to make a mutation?
This is an insert
operation on table users
.
The payload for each event is mentioned here
Now we are going to capture this insert operation to trigger our event.
SendGrid SMTP Email API
For this example, we are going to make use of SendGrid
's SMTP server and use nodemailer
to send the email.
Signup on SendGrid and create a free account.
Create an API Key by following the docs here
Write the webhook
const nodemailer = require('nodemailer');const transporter = nodemailer.createTransport('smtp://'+process.env.SMTP_LOGIN+':'+process.env.SMTP_PASSWORD+'@' + process.env.SMTP_HOST);const fs = require('fs');const path = require('path');const express = require('express');const bodyParser = require('body-parser');const app = express();app.set('port', (process.env.PORT || 3000));app.use('/', express.static(path.join(__dirname, 'public')));app.use(bodyParser.json());app.use(bodyParser.urlencoded({extended: true}));app.use(function(req, res, next) {res.setHeader('Access-Control-Allow-Origin', '*');res.setHeader('Cache-Control', 'no-cache');next();});app.post('/send-email', function(req, res) {const name = req.body.event.data.new.name;// setup e-mail dataconst mailOptions = {from: process.env.SENDER_ADDRESS, // sender addressto: process.env.RECEIVER_ADDRESS, // list of receiverssubject: 'A new user has registered', // Subject linetext: 'Hi, This is to notify that a new user has registered under the name of ' + name, // plaintext bodyhtml: '<p>'+'Hi, This is to notify that a new user has registered under the name of ' + name + '</p>' // html body};// send mail with defined transport objecttransporter.sendMail(mailOptions, function(error, info){if(error){return console.log(error);}console.log('Message sent: ' + info.response);res.json({'success': true});});});app.listen(app.get('port'), function() {console.log('Server started on: ' + app.get('port'));});
Deploy
Environment variables
After remixing to your own project on Glitch, modify the .env
file to enter the
SMTP_LOGIN
,SMTP_PASSWORD
,SMTP_HOST
values appropriately.
Additionally, you should also configure the sender and receiver address using
SENDER_ADDRESS
RECEIVER_ADDRESS
env variables.
Congrats! You have written and deployed your first webhook to handle database events.
Build apps and APIs 10x faster
Built-in authorization and caching
8x more performant than hand-rolled APIs
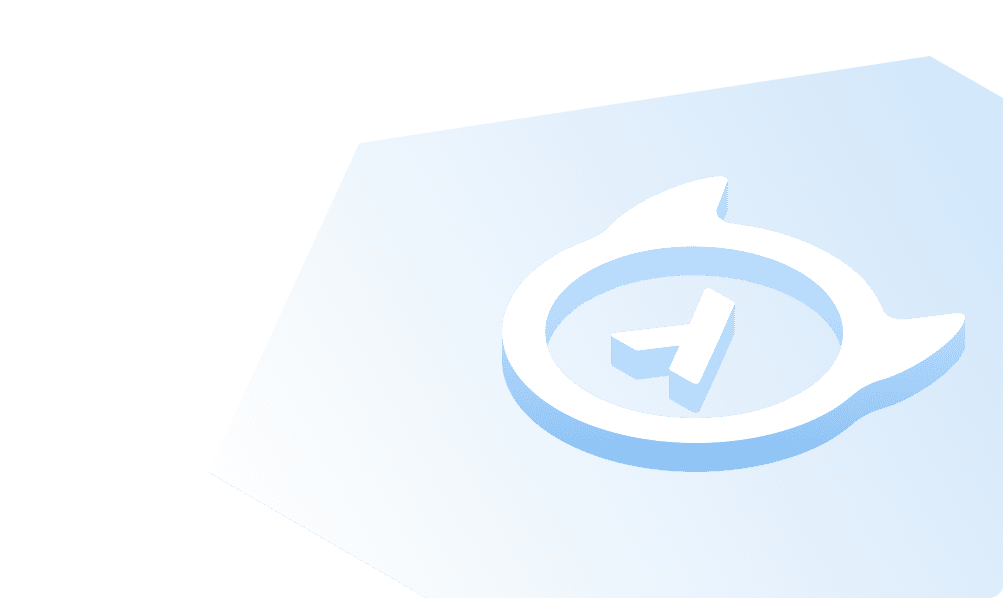
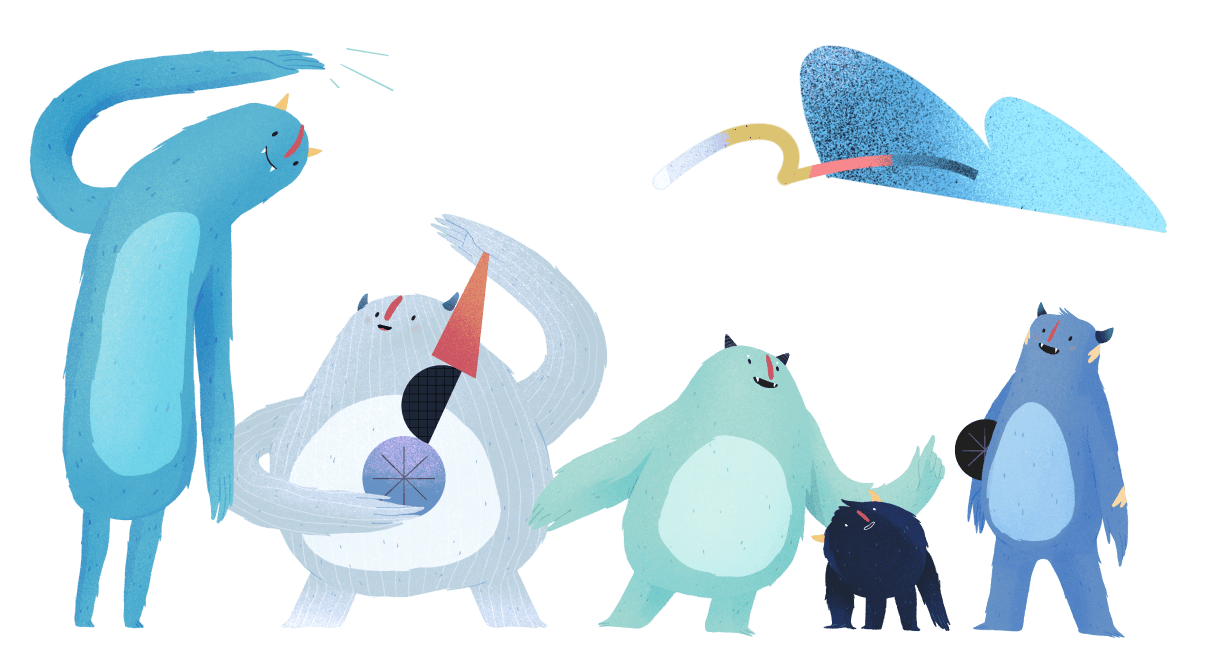